안드로이드/Jetpack Compose
[안드로이드] Compose - Scaffold, Row, Column
안드뽀개기
2022. 4. 21. 22:47
반응형
Compose는 플러터랑 매우 비슷하다..
이전에 플러터를 책 두권을 떼고 선언형 UI를 처음 접했고, 큰 재미를 느꼈다.
하지만.. 현업에 바빠서 배움이 미뤄지길래 지금이라도 Jetpack Compose를 공부하고자 한다.
Empty Compose 프로젝트를 생성하면 이전과 동일하게 액티비티가 생성된다.
액티비티에 setContent { } 안에 내용이 화면이 되는 것이다.
@Composable 어노테이션이 붙여서 UI 코드를 작성할 수 있고,
@Preview 어노테이션을 붙이면 오른쪽에 미리보기가 생성된다.
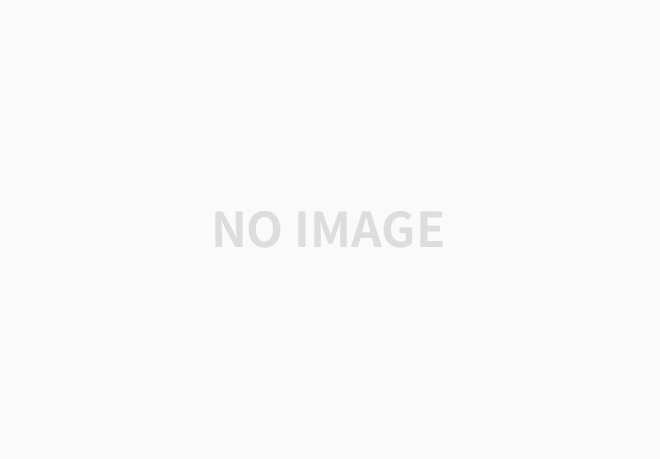
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
class MainActivity : ComponentActivity() { | |
override fun onCreate(savedInstanceState: Bundle?) { | |
super.onCreate(savedInstanceState) | |
setContent { | |
TestTheme { | |
// A surface container using the 'background' color from the theme | |
Surface(modifier = Modifier.fillMaxSize(), color = MaterialTheme.colors.background) { | |
Greeting("Android") | |
} | |
} | |
} | |
} | |
} | |
// 뷰 | |
@Composable | |
fun Greeting(name: String) { | |
Text(text = "Hello $name!") | |
} | |
@Preview(showBackground = true) | |
@Composable | |
fun DefaultPreview() { | |
TestTheme { | |
Greeting("Android") | |
} | |
} |
Scaffold
Scaffold는 플러터에서 마찬가지로 지원해주는데, 이는
머티리얼 디자인을 사용할 수 있게 해준다.
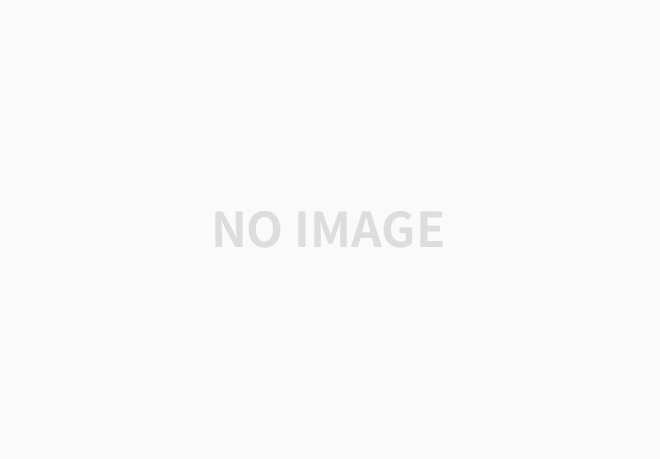
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// 뷰 | |
@Composable | |
fun Greeting(name: String) { | |
Scaffold( | |
topBar = { TopAppBar(title = { Text("TopAppBar")},backgroundColor = Color.Blue, contentColor = Color.White, )}, | |
floatingActionButtonPosition = FabPosition.End, | |
floatingActionButton = { | |
FloatingActionButton(onClick = {}, backgroundColor = Color.Red, contentColor = Color.White ) { | |
Text(text = "클릭") | |
} }, | |
) { | |
Text(text = "Hello $name!") | |
} | |
} |
Scaffold를 이용하여 topBar와 floatingActionButton을 화면에 표시했다.
Row와 Column
Row와 Column은 가로 및 세로로 뷰를 그릴때 사용한다.
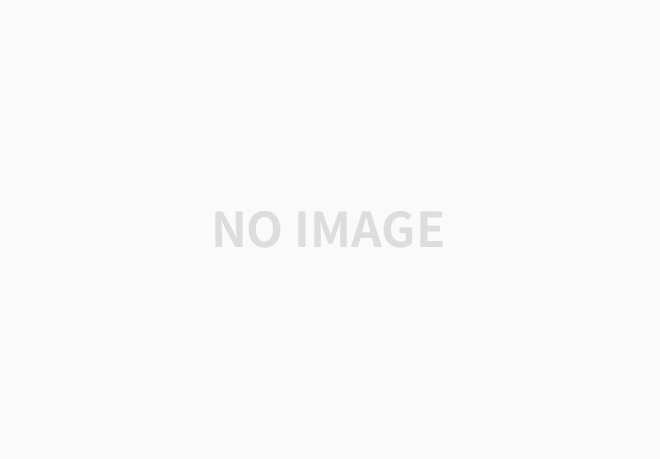
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// 뷰 | |
@Composable | |
fun Greeting(name: String) { | |
Scaffold( | |
topBar = { TopAppBar(title = { Text("TopAppBar")},backgroundColor = Color.Blue, contentColor = Color.White, )}, | |
floatingActionButtonPosition = FabPosition.End, | |
floatingActionButton = { | |
FloatingActionButton(onClick = {}, backgroundColor = Color.Red, contentColor = Color.White ) { | |
Text(text = "클릭") | |
} }, | |
) { | |
MyComposableView() | |
} | |
} | |
@Composable | |
fun MyComposableView(){ | |
Column( | |
Modifier | |
.background(color = Color.Gray) | |
.verticalScroll(rememberScrollState())) { | |
for (i in 0..30) { | |
rowComposableView() | |
} | |
} | |
} | |
@Composable | |
fun rowComposableView(){ | |
// 호리젠탈 리니어 레이아웃 | |
Row( | |
Modifier | |
.padding(10.dp) | |
.background(Color.Yellow), | |
verticalAlignment = Alignment.CenterVertically | |
){ | |
Text(text = "안녕하쇼", | |
Modifier | |
.background(Color.Green) | |
.padding(all = 10.dp)) | |
Spacer(modifier = Modifier.size(10.dp)) | |
Text(text = "안녕하쇼") | |
Spacer(modifier = Modifier.size(10.dp)) | |
Text(text = "안녕하쇼") | |
} | |
} |
Column안에 30개의 Row를 그렸다.
Modifier : 배경, 패딩 등을 설정
Spacer : 뷰 사이의 간격을 설정할 수 있는 위젯
Column의 Modifier.verticalScroll(rememberScrollState()) 속성 : Column이 세로 스크롤이 가능하도록 하는 속성
반응형