이번엔 버튼 속성에 대해 알아보겠습니다.
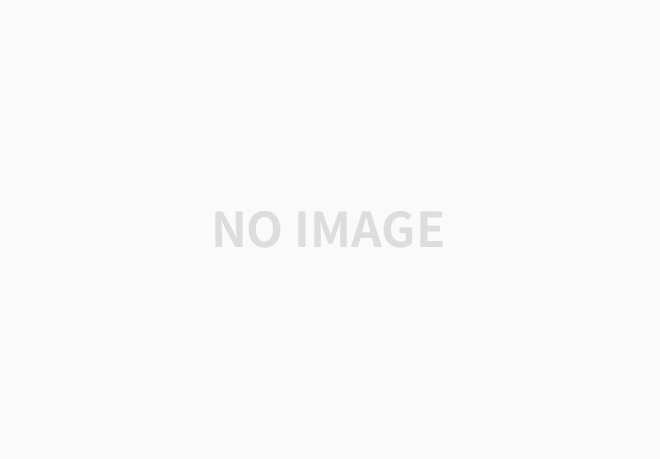
버튼 1
elevation : 버튼이 z축으로 올라와 보이는 효과
- defaultElevation : elevation의 기본값
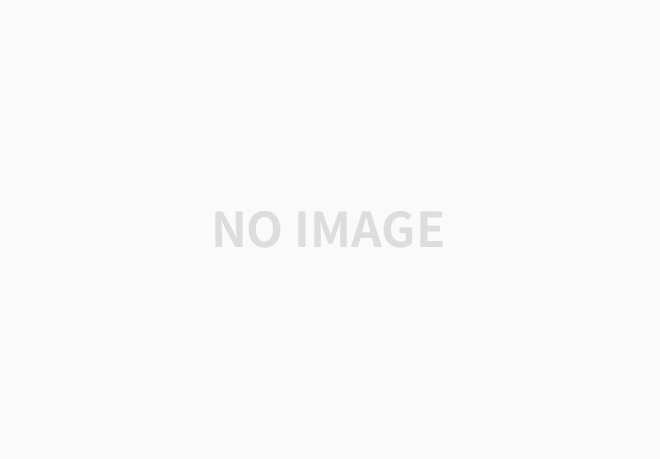
버튼2
elevation : 버튼이 z축으로 올라와 보이는 효과
- defaultElevation : elevation의 기본값
- pressedElevation : 버튼이 눌렸을 때 elevation 값,
- disabledElevation : 버튼의 enabled가 false일 때의 elevation 값
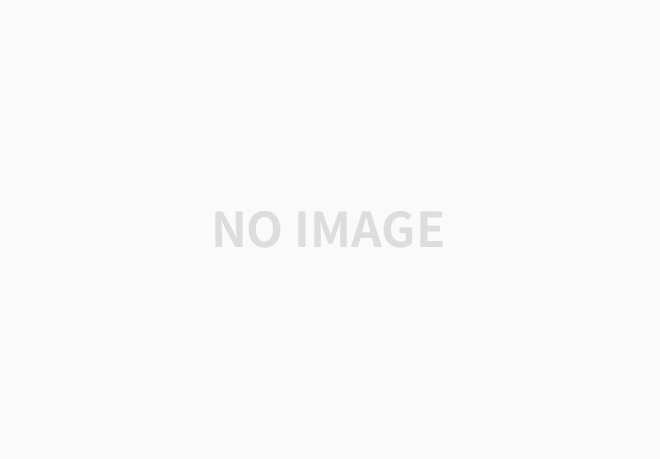
버튼3
shape : 버튼의 모양
- CircleShape
- RectangleShape
- RoundedCornerShape(dp)
border : 테두리의 색, 두께 지정
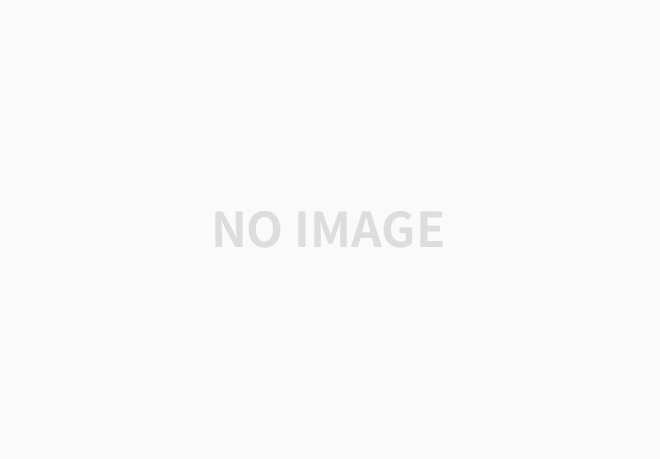
버튼4
border : 테두리의 색, 두께 지정
- 테두리의 색을 그라데이션으로 줄 수 있다.
colors : 버튼 색상을 설정
- backgroundColor : 기본 배경색 설정
- disabledBackgroundColor : enable이 false일 때의 배경색 설정
interactionSource : 사용자의 interaction에 대한 설정
- remeber { } 를 이용하여 사용자의 interaction이 변경되었을 때, UI를 다시 그린다
- interactionSoucre.collectionIsPressedAsState()를 통해 사용자가 버튼을 눌렀을 때 isPressed 변수에는 true값이 저장, 버튼을 뗐을 때에는 false값이 저장된다
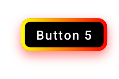
버튼5
버튼 4에서 그림자를 추가한 버튼입니다.
modifier : 뷰의 높이, 너비, padding, 등 다양한 설정 가능
여기서는 Modifier의 extension 함수를 만들어 커스텀 그림자를 적용
커스텀 그림자의 소스는 하단에 링크 참조
https://smashandroid.tistory.com/88
shadowRadius : 버튼이 눌렸을 때 그림자가 사라지고, 버튼을 뗐을 때 버튼이 나타나도록 설정하기 위한 변수
shadowRadusWithAnim : 그림자가 사라지거나 나타날때의 애니메이션을 적용하기 위한 변수
'안드로이드 > Jetpack Compose' 카테고리의 다른 글
[안드로이드 Compose] 버튼 Custom Shadow (0) | 2022.04.24 |
---|---|
[안드로이드 Compose] AutoResizeText (0) | 2022.04.24 |
[안드로이드] Compose - LazyColmn (0) | 2022.04.22 |
[안드로이드] Compose - Scaffold, Row, Column (0) | 2022.04.21 |